In HTML5 & CSS: The Basics, we discussed how to display web page text in a particular font but remember that the font has to exist on the user's computer. The variability of fonts on different users' computers is why we assign fonts in families.
Alternatively, we can upload web fonts to our web server (just like we upload an image or style sheet), link to them in the code, and ensure that anyone viewing our site will see it in those fonts.
Using Custom Fonts
A lot of brand guidelines require the use of a particular font. For example, the IU brand guidelines require that, for a serif font, we use Georgia Pro. The university owns a license to the font to allow it to be used on the web. We will be using Georgia Pro for our headings.
Here is an example of the CSS required to create a custom web font using the IU serif font, Georgia Pro:
@font-face {
font-family: "GeorgiaPro";
src: url("../fonts/Georgia-Pro/georgia-pro.eot") format("embedded-opentype"),
url("../fonts/Georgia-Pro/georgia-pro.woff") format("woff"),
url("../fonts/Georgia-Pro/georgia-pro.ttf") format("truetype"),
url("../fonts/Georgia-Pro/georgia-pro.svg#GeorgiaPro") format("svg");
}
Exiting code block.
The @font-face rule requires that we give the font a name using the font-family property. This name can be whatever we want it to be, but should be descriptive because it's the name that we'll be using to refer to the font in our style sheet. We are also required to include at least one src property. The src property is similar to the src attribute for an image; it defines the location of the source file or files for the display of the font. The src property takes a url() and has an optional format() definition as well. This tells the browser what type of font to expect.
In our example, there are four types of font files that are embedded: EOT, WOFF, TTF, and SVG. If you want more information, w3schools has a great article about web fonts.
Let's add Georgia Pro to our site for use in headings. The font files are located within the fonts folder inside the Code the Web folder.
The first step is to add the font to our style sheet.
Step1. If necessary, activate htmsw.css in the code editor.
Step2. To add Georgia Pro to our style sheet, at the bottom of the file, type the code in bold below [Region 24]:
/* FONT PATHS
* -------------------------- */
@font-face {
font-family: "GeorgiaPro";
src: url("../fonts/Georgia-Pro/georgia-pro.eot") format("embedded-opentype"),
url("../fonts/Georgia-Pro/georgia-pro.woff") format("woff"),
url("../fonts/Georgia-Pro/georgia-pro.ttf") format("truetype"),
url("../fonts/Georgia-Pro/georgia-pro.svg#GeorgiaPro") format("svg");
font-style : normal;
font-weight: normal;
}
Exiting code block.
Step3. To change the font family for our headings, at the top of our CSS file, type the code in bold below [Region 26]:
h1, h2, h3, h4, h5, h6 {
font-family: 'GeorgiaPro', serif;
letter-spacing:.13rem;
}
Exiting code block.
Step4. Save htmsw.css and refresh index.html.
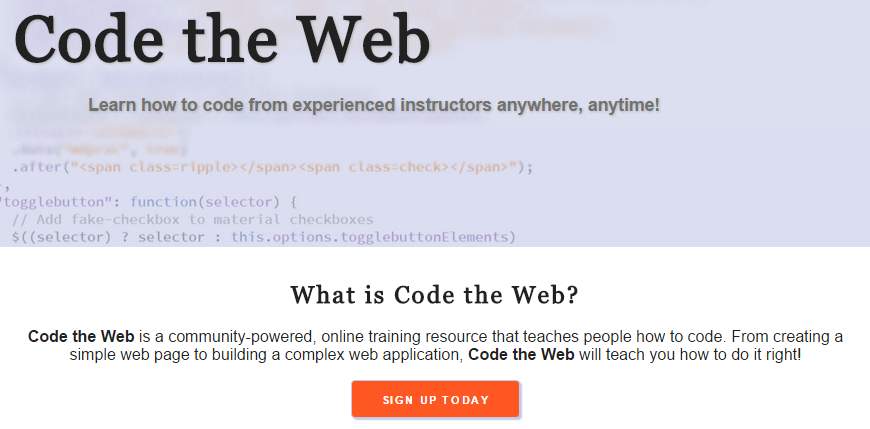
Using Font Repositories
Another way that we can embed fonts into a web page is by using a font repository. Font repositories host web fonts online that can be embedded without uploading the font files to our web server and writing lots of CSS code. One of the most popular font repositories is Google Fonts. We will be using Google Fonts today to add another font to our page that we want for everything except headings.
Step1. If necessary, switch to the web browser.
Step2. To open a new tab, press:
Control key+t
Step3. To navigate to Google Fonts, in the web browser's address bar, type:
https://www.google.com/fonts, press: Enter
NOTE: You can use whichever font you would like in today's example.
Step4. To search for the Raleway font, in the search field, type:
Raleway, press: Enter
Step5. To add Raleway to our collection,
Click
Step6. To review our collection, in the upper right of the Collection panel,
Click
Tab | Function |
---|---|
Specimen | Explore how the selected font looks and behaves in several different elements, weights, and styles. |
Styles | Browse through all the font styles side-by-side. |
Test Drive | See how the font looks in many different elements in a page-like mockup. |
Character Set | Explore the character sets available in the selected font. |
Compare | Compare the font character by character to another font. |
Description | A prose description of the font with credits to the font designers, links to documentation, information about the font's license, and possibly links to related fonts. |
Step7. To begin implementing the font into our site,
Click
Adding the Font to our Style Sheet
We will be using the import functionality in CSS. Importing in CSS is a way to include the CSS from another file into a site or project. Its syntax is as follows:
@import url(path/to/css/resource.css);
Exiting code block.
This functions much in the same way as includes or imports in other languages, bringing the entire contents of the CSS resource into the file that specifies the import.
We will use @import to add the Raleway font to our pages.
Step1. If necessary, activate htmsw.css in the code editor.
@import url(https://fonts.googleapis.com/css?family=Raleway);
body {
color: #212121;
}
Exiting code block.
Step2. To add Raleway as the default font in our site, in our style sheet, type the code in bold below [Region 28]:
body {
color: #212121;
font-family: 'Raleway', sans-serif;
}
Exiting code block.
Step3. Save htmsw.css and refresh index.html.
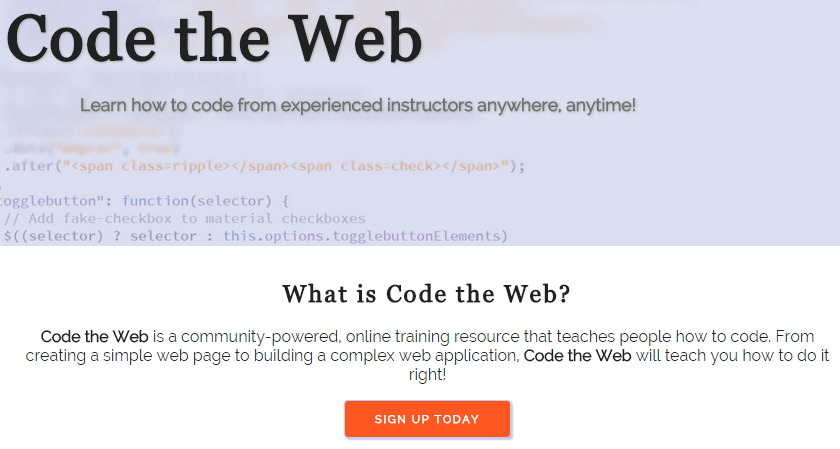
Importing Using the Link Element
If a font is only to be used on a single page in a site, it's best to use the <link> element provided on the Standard tab in step 3 of the Use tools. This ensures that the font resource is only loaded when it's needed. In our case, since we're using the font on every page, placing it in our global style sheet is the best course of action.
Generally, for each resource that is loaded from another location, the page load will take longer. This is the reason behind using <link> if the font is only needed on one page vs. using @import in the style sheet.
Now that we have squared away the structure and fonts of our site, let's look at embedding video in a web page.